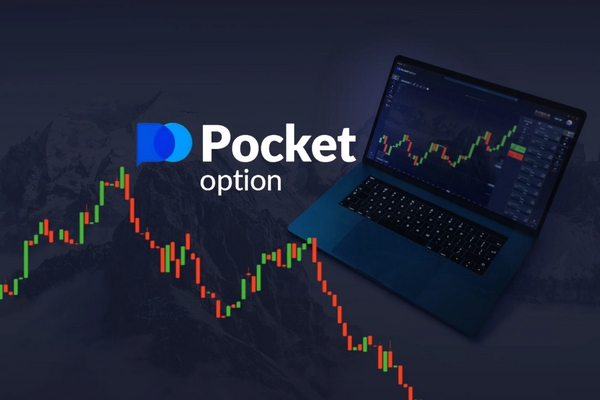
Understanding the Pocket Option API in Python for Trading Success
In the world of online trading, the ability to automate strategies and access real-time market data is essential for success. The pocket option api python pocket option api python has emerged as a powerful tool for traders who want to leverage Python’s programming capabilities to enhance their trading endeavors. This article will guide you through the various features of the Pocket Option API, how to set it up, and best practices for written code, enabling you to derive maximum benefit from your trading activities.
What is the Pocket Option API?
The Pocket Option API is a set of programming interfaces provided by Pocket Option, a popular trading platform, which allows developers to build applications that interact with their trading infrastructure. The API facilitates automated trading, access to real-time market data, historical trading data, and account management functionalities, empowering traders to create custom trading solutions tailored to their strategies.
Getting Started with the Pocket Option API
To start using the Pocket Option API with Python, you need to follow a few necessary steps. First, ensure that you have an account on Pocket Option and have registered for API access. Once you have your API key, you can begin the coding process. Install the necessary libraries if you haven’t yet:
pip install requests
The “requests” library is imperative for making HTTP requests to the API endpoints, making your interaction with the Pocket Option platform much smoother.
Authenticating Your API Requests
The first step when using the Pocket Option API is to authenticate your requests using your API key. Here’s an example of how you can achieve this:
import requests
API_KEY = 'your_api_key_here'
URL = 'https://api.pocketoption.com/v1/your_endpoint'
headers = {
'Authorization': f'Bearer {API_KEY}',
}
response = requests.get(URL, headers=headers)
data = response.json()
print(data)
This code snippet sends a GET request to a specified endpoint, utilizing the API key for authentication and seamlessly processing the response.
Fetching Market Data
One of the key functionalities of the Pocket Option API is the ability to fetch market data. You can retrieve information about various assets, including their current price, volatility, and trading volume. Here is an example of how to fetch market data:
def get_market_data():
response = requests.get('https://api.pocketoption.com/v1/market_data', headers=headers)
market_data = response.json()
return market_data
market_info = get_market_data()
print(market_info)
Executing Trades
Executing trades programmatically is another powerful feature of the Pocket Option API. The following example demonstrates how to place a trade:
def place_trade(asset_id, amount, direction):
url = 'https://api.pocketoption.com/v1/trade'
payload = {
'asset_id': asset_id,
'amount': amount,
'direction': direction,
}
response = requests.post(url, headers=headers, json=payload)
result = response.json()
return result
trade_result = place_trade(asset_id='12345', amount=10, direction='call')
print(trade_result)
In this example, the function places a trade for a specified asset with a defined amount and direction (call or put).
Managing Your Account
Apart from trading functionalities, the Pocket Option API also allows you to manage your account effectively. You can access account balances, transaction history, and other vital information. Here’s how to retrieve account balance:
def get_account_balance():
response = requests.get('https://api.pocketoption.com/v1/account/balance', headers=headers)
balance_info = response.json()
return balance_info
account_balance = get_account_balance()
print(account_balance)
Best Practices for Using the Pocket Option API
To make the most of the Pocket Option API in Python, consider the following best practices:
- Handle exceptions: Always incorporate error handling into your API calls to manage unexpected responses or failed requests.
- Respect rate limits: Ensure you are aware of the API’s rate limits to avoid being temporarily blocked from making requests.
- Documentation: Refer frequently to the official Pocket Option API documentation for updates or changes in endpoints and functionalities.
- Testing: Test your scripts thoroughly in a controlled environment before deploying them in a live trading scenario.
Conclusion
The Pocket Option API, when utilized effectively with Python, can significantly enhance your online trading capability. From automating trades to retrieving pivotal market data, the possibilities are expansive. By following the outlined steps and best practices, you can set up a robust trading algorithm that maximizes your market opportunities. With continuous learning and adjustment of your strategies, the Pocket Option API can be your gateway to trading success.